Angular Interview questions for 2021
Our guide with questions and answers to help you prepare for Angular interviews
Angular Interview tips
First off— if you have passed the first stage of your developer interview process, congratulations! Let’s get you prepared for the next stage!
Angular Interview - Step 1:
In general, companies looking to hire Angular developers use the following 3 approaches to interview candidates:
1. The technical interview — this involves technical questions relating directly to angular asked in a face-to-face interview.
2. The technical task — a frontend task to demonstrate your prowess. This is usually a PDF sent after an initial interview.
3. The “automated” task — some companies will send over a task before a first interview. These consist of a coding task that needs to be done in the browser. This approach is generally taken by large companies, presumably due to a large number of applicants
The technical interview
Interviewers usually ask a wide range of Angular questions in this category. The following Q&A groups questions which are generally asked together:
Architecture
What are the different ways one can send and receive data between components? Are services singletons?
Services as well as Input() and Output() properties can be used to transfer data between components. Services are singletons by default, but with module lazy loading you can end up with several instances of a service.
What are Pipes? What are the drawbacks of using the async pipe in your template? What is the difference between pure and impure pipes?
Pipes are a simple way to transform values in Angular. Using the async pipe multiple times in the template creates multiple subscriptions to the same observable, which can cause performance issues.
A pure pipe is only called when angular detects a change in the value, an impure pipe is called on every change detection cycle.
How do you handle translations?
Internationalization (i18n) can be added to your app to support multiple locales. Integrating i18n can make sure that locales match for decimals and dates, and also add support for text translations and word pluralizations.
What are directives?
A directive changes the appearance or behavior of elements in the DOM. There are 3 types of directives:
1. Components — these are directives with a template
2. Structural directives — these change the DOM layout by adding and removing DOM elements (ex: NgFor, NgIf)
3. Attribute directives — these change the appearance and behavior of an element, component or another directive (ex: NgClass, NgModel)
What are decorators?
Decorators are functions that are always prefixed by an “@” symbol. Some common decorators are the @component, @module and @HostListener
What is tree-shaking?
Tree-shaking is the process of removing unused code from your build. This makes your build significantly smaller.
What is transpiling? What are the available compilers in Angular 9 and above?
Transpiling is the process of converting typescript to javascript. There are 2 compilers in Angular: the AOT (ahead of time) and the JIT(just in time) compiler. The AOT compiler converts the HTML and Typescript code into Javascript before your browser downloads the code in your production build. The JIT compiler compiles your app in the browser at runtime and is mainly used during development. The compilers are necessary as browsers can’t understand templates directly.
What are service workers and PWAs?
The default service worker in Angular acts as a caching mechanism for your Angular apps. They function as a proxy and intercept all incoming and outgoing HTTP requests. They are also preserved after a user closes the tab. Using a service worker in your app is the first step to making your app a PWA, which you can read about in more detail in our post .
What are HTTP interceptors? How are service workers and HTTP Interceptors different?
Http Interceptors inspect and modify incoming and outgoing requests in your application. Http interceptors only listen to requests from the HttpClient provider, while service workers intercept all requests (including assets and fetch requests).
What’s the lifecycle of a component?
If you haven’t already, memorize this diagram:
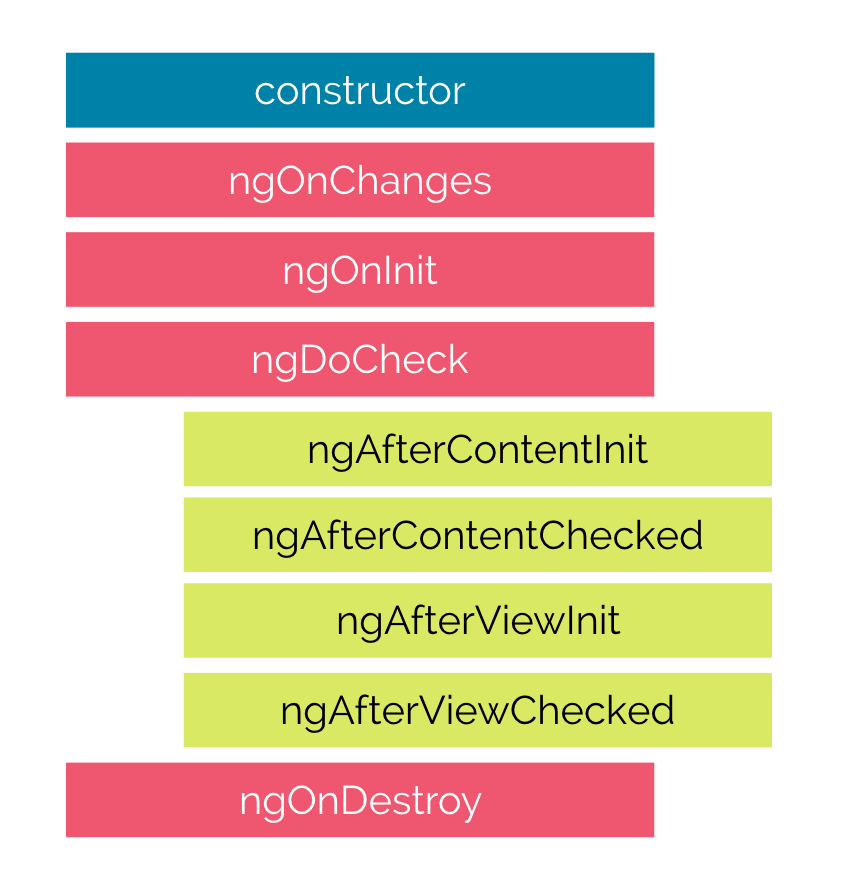
Component lifecycle
How do you get sub-children? What is the difference between ViewChild and ContentChild? In which part of the lifecycle can you read use ViewChild?
Projected content can be accessed with ContentChild, while DOM elements and components directly in the template can be accessed with viewchild. ViewChildren can be used in ngAfterViewInit, since it wouldn’t be initialised before this point.
What is ViewEncapsulation? What are the available viewEncapsulation properties and how do they work?
ViewEncapsulation defines whether the template and styling in the component effects the whole application. There are 3 main options for this:
1. None: Styles and templates are global.
2. Emulated: Uses generated string attributes to make the styles unique to the component and adds the new host element attribute to all selectors.
3. ShadowDom: Uses the Shadow DOM to encapsulate the styles.
What are the available ChangeDetection strategies? How do you re-trigger a change detection within the component?
Angular uses zone.js internally to detect any changes to the DOM. In the component decorators, you can define a custom ChangeDetection to make your component more efficient (by skipping over change detection runs with no changes).
There are 2 main options for changeDetection:
1. Default: The default changeDetection strategy. This is the friendliest for beginners, but can cause unnecessary cycles and can make the app feel more sluggish.
2. OnPush: No change detection cycles are run unless the inputs change. A common pitfall to using this is that any values passed to the component need to be immutable, or changes won’t be detected.
You can call markForCheck() or detectChanges(). DetectChanges will run change detection immediately, while markForCheck() will not run change detection, but will mark its parents as needing change detection (so it waits for the next cycle)
What’s the difference between ng-template, ng-container and ng-content?
ng-content: This is used to project content into your component. This means that you can avoid repeating parts of your template by doing this (courtesy of codeburst.io ):
<app-wrapper>
<div class="error" style="background-color: green; padding: 20px">
Child Error
</div>
<div class="warning" style="background-color: green; padding: 20px">
Child Warning
</div>
</app-wrapper>
<div style="background-color: red; padding: 20px">
<ng-content select=".error"></ng-content>
</div>
<div style="background-color: orange; padding: 20px">
<ng-content select=".warning"></ng-content>
</div>
ng-container: This is used to group content without effecting your DOM structure. This is useful when you want to write 2 structural directives at on the same element
ng-template: This is used as a “temporary” element that allows you to add directives and binds children to the parent. If the code is not referenced, it will not be shown in the end template. This may seem useless, but it can be used as a reference in the else clause:
<div>
Hello word!
<div *ngIf="false else content">
Shouldnt be displayed
</div>
</div>
<ng-template #content>
Should be displayed
</ng-template>
What is lazy loading? What are the different types of lazy loading?
By default, all modules are eagerly loaded — that means that parts that are not visible to the user within the page are all loaded in the beginning. This causes a high TTI (time to interactive) and can in turn reduce your overall SEO score.
There are 3 main types of lazy loading achievable within your browser:
1. Image lazy loading — this is not angular specific, but images can be loaded lazily by adding the loading=lazy
attribute to them. This way, off-screen images are deferred until the user scrolls to them
2. Module lazy loading — with this lazy loading, each module is it’s own bundle and has it’s own route. When the user navigates to a new “module route”, the relevant module is loaded
3. Component lazy loading — this was very recently added and allows you to lazy load specific components — however the architecture for this can get messy.
What’s new in Angular/What has been added recently?
In Angular 9, the new Ivy engine was added. Angular 10 dropped support for older browsers in their polyfills, and now has a new build configuration (“ — strict”) to force better typescript practices. It also added quality of life changes like the upgrade to Typescript 3.9 and type checking improvements to the compiler-cli.
What are “declarations”, “imports” and “bootstrap” in ngModule?
- Declarations are used to declare components that are available within the module.
- Imports are the required modules that are needed by the module
- The bootstrap array is used to define the components to be created. This is usually done to define entryComponents
What are router guards? What is the difference between canActivate and canLoad?
Router guards are used to define access permissions to specific routes. The canLoad authguard prevents modules from being loaded, while canActivate stops navigation but still loads the module.
RxJs
What operators do you use most frequently? Please describe how they work?
Pick an operator that is both complicated and interesting but still easy enough to explain. CombineLatest or Merge, for example. http://learnrxjs.io/ is a great resource on learning these operators.
What is a common pitfall of subscriptions in angular?
Subscriptions always need to be unsubscribed. Usually, this is done using the take operator in OnDestroy, but there are libraries such as ng-neat that provide an untilDestroy
operator.
How do you debug pipes?
Tap with a debugger inside is a good way to debug pipes, but can get messy. A neater way is to use rxjs-spy , a debugging tool for all your pipe plumbing needs!
Styling (SCSS)
How do you reduce code repetition (in styles)?
For loops can be used in styles, although they may end up generating a lot of CSS. If statements can also be written directly in SCSS. Mixins can be used for more generic code snippets.
What are mixins?
To quote the docs: “Mixins allow you to define styles that can be re-used throughout your stylesheet. They make it easy to avoid using non-semantic classes like .float-left
, and to distribute collections of styles in libraries”
Other notes
For this type of interview, it is important to prepare your real-life environment by:
- Turning off your landline/phone
- Take a good look at your background.
- Making sure you have sufficient warm lighting
- Making sure that the camera angle is favourable
- Ensuring your webcam settings don’t make you look overexposed (too bright/too much contrast).
Keep in mind that you are competing against other candidates, so the little details stand out!
Preparing for the “technical” task
Testing how candidates fail
Bear in mind that there are plenty of companies that are more interested in testing how candidates fail their task, and how they handle stressful situations than they are in the output of the task. Some companies may purposefully give you a two-week task to finish in a few days. If you are confident that this is the case — don’t panic. Focus and prioritise the most important features first.
What your interviewer wants to see
When working on your technical task, put yourself in the shoes of your interviewer for a moment and think about what they are on the lookout for. This may be:
- Best practices (Check out the Angular Styleguide or the TypeScript handbook ). You can also set up the project using
strict
mode using this guide , and look into common angular best practices, such as using trackBy with ngFor, which immediately tells the interviewer you care about performance. - Knowledge of testing, how to test and what to test.
- Code style, and how it fits with the rest of the team.
- Can you write cleaner asynchronous code by using and chaining rxjs operators?
- The interviewer will also be on the lookout for soft skills, such as how fast you can pick up something new, how you may get along with the team, how you manage your time and how you organise your tasks, so be sure to have this prepared. These are questions that may be asked before or after your technical task.
Preparing your environment
This may sound obvious, but you need to prepare your machine for technical tasks. You should also try to give accurate time estimates on your task. In the case of Angular, make sure your global CLI version is at the latest version, and make sure you have the correct version of Node installed. I also recommend installing a rough mockup tool like Balsamiq — more on this in a bit.
You can also initialize the project, and make sure it builds. If you were interviewed on a specific topic before this technical task, make sure that the libraries are also installed and ready to use (ex: Bootstrap/Angular Material, NgRx, etc.) You should also add some base modules and structure to your project before receiving the task, and remove all placeholder text to give yourself a head start.
After you receive the task
After you receive your task — take a deep breath. Then, underline and prioritize the specific project requirements. Then, create a quick mockup of what you expect the final system to look like — this is extremely helpful for your architecture (splitting of the component/module). This is where balsamiq comes in. If you are unfamiliar with mockup tools, feel free to draw on a piece of paper (ideally in pencil, with a ruler). You should also spend a few minutes before starting the task thinking about any tricks within it.
Preparing for the “automated” task
Before the test
It is rare to see automated interview tasks which are specifically Angular-related. To prepare for this task, you need to brush up on your Searching and Sorting algorithms, and you also need to practice your “BigO analysis skills” to optimize your coding snippets.
Hackerrank has some excellent videos on the topics, I highly recommend you read up on these topics:
General optimisation:
- Big O: https://www.youtube.com/watch?v=v4cd1O4zkGw
- LinkedList: https://www.youtube.com/watch?v=njTh_OwMljA
Sorting:
- Quicksort: https://www.youtube.com/watch?v=SLauY6PpjW4
- Bubblesort: https://www.youtube.com/watch?v=6Gv8vg0kcHc
- Mergesort: https://www.youtube.com/watch?v=KF2j-9iSf4Q
Searching:
- Binary search: https://www.youtube.com/watch?v=P3YID7liBug
- Graph search/BFS/DFS: https://www.youtube.com/watch?v=zaBhtODEL0w
You should also practice the video’s content, then graduate to code challenges on platforms like Codewars or HackerRank .
These automated interview tasks will also (generally) rule out candidates that don’t finish a solution in time, so the best approach for these is to write unoptimised code as quickly as possible, then optimize its speed as well and add comments/linting if you still have time.
On some platforms, you can’t submit any code — they are only interested in the answer. For this reason, be sure to prepare a local environment with Node and your editor of choice. You should also make sure to configure your IDE for debugger tooling.
Unfortunately, these tests also usually have a JQuery section, so be sure to revise it beforehand. Be sure to stick to the latest ES syntax when coding your answer as well.
During/after the test
Usually, the primary goal of automated tests is to test out your thinking skills. If you see only a few minutes left on the timer, leave comments on what you were trying to do — this might get you through the first stage. Some coding tests also track your changes in real time, so it’s also a good idea to use the browser editor — rather than pasting your code in from an external editor (or worse, a readymade solution).
Top Angular Interview Questions - Conclusions
This goes without saying, but good luck with your interviews! Always remember to put yourself in the shoes of your interviewer and why you are being asked a particular question.